Dictionaries
A Dictionary is a container for data organized into key-value pairs. A key is always a symbol, but the value can be anything, including a number, symbol, list, or even another dictionary. You can create and manage dictionaries using the dict object, and the associated family of dictionary manipulation objects. Like arrays and strings, dictionaries have names and can be passed between objects with messages like dictionary u12345678
.
For developers, a dictionary is most similar to a JSON object, and there are convenience functions in the API for converting a dictionary to a JSON string, as well as updating the contents of a dictionary with JSON.
When to Use Dictionaries
Dictionaries are useful for working with structured data, especially when that data has a hierarchical or labeled format. Imagine you were trying to simulate a large number of agents, each one modeling a simple creature with some kind of behavior. The state of each creature might look something like the following.
{
"position": {
"x": 2,
"y": 5
},
"mood": "nostalgic"
}
With a dictionary, the values of your object not only have a place in a hierarchy, they also have labels.
Working with Dictionaries
Create a new dictionary using the dict object. The argument for the dict object is the unique name for the dictionary. If you do not give the dictionary a name, Max will generate a unique name automatically. Two dict objects with the same name will reference the same dictionary.
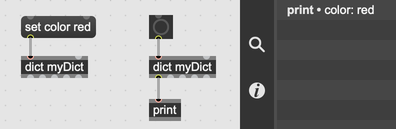
If you use a message object to display a string, you'll see the actual values that pass between Max objects when sending a dictionary in a message. In a message, a dictionary is represented by the symbol dictionary
, followed by the unique name of the dictionary.
Editing a Dictionary
Edit the contents of a dictionary by double-clicking on a dict object, or by
sending the dict object the edit
message. This will open a text editor for modifying the contents of a dictionary.
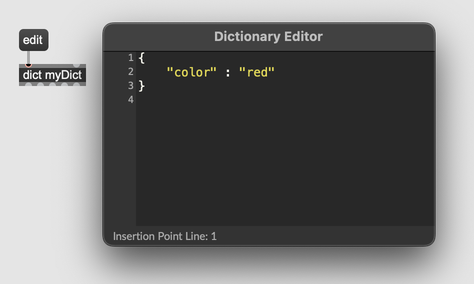
Dictionaries can be edited using JSON syntax (except that dictionaries don't support boolean (true
/false
) values). Keys must be strings, enclosed in quotation marks. Values can be numbers, strings, arrays (denoted with square brackets) or dictionaries (denoted by curly braces). Since the entire structure is itself a dictionary, curly braces must enclose the whole dictionary expression.
By sending the export
message to the dict object, you can write the contents of a dictionary to a file, in either JSON or YAML format. Using the import
message, you can then read such a file into a dict object.
pattr and pattrstorage
The pattr and pattrstorage objects will store the contents of a dictionary. Because a dictionary is not a simple numeric value, you can't interpolate between dictionary values using floating-point values for recall
. However, in other respects, dictionaries are fully compatible with pattr and pattrstorage.
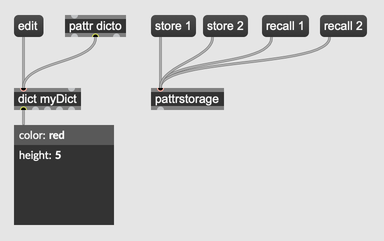
JavaScript
Use the Dict
class to create a JavaScript reference to a Max Dictionary. The name of the dictionary will be the first argument to the constructor, or an automatically generated unique name if none is provided. If the js
object is in a patcher that contains a dictionary with the same name, then the js
Dict
object and the Max dict object refer to the same dictionary. Modifying the contents of one will change the contents of the other.
var max_dict = new Dict("mydict");
To manipulate the contents of the dictionary, use the get
and set
methods.
max_dict.set("color", "red");
console.log(max_dict.get("color")); // prints "red"
The parse
method can initialize the contents of a dictionary using a JavaScript JSON serialization. This is a useful way to convert a JavaScript object to a Max dictionary.
let obj = {x: 1, y: 2};
let serial = JSON.stringify(obj);
max_dict.parse(serial); // dictionary now has the keys x and y with values 1 and 2
To receive a dictionary in a JavaScript function, define a function named dictionary
.
The first argument to this function will be the name of the dictionary.
function dictionary(dict_name) {
var myDict = new Dict(dict_name); // now "myDict" links to the passed-in string.
}
To send a Max dictionary out of an outlet defined in JavaScript, send the symbol "dictionary" followed by the name of the dictionary.
function bang() {
var myDict = new Dict();
myDict.set("wood", "balsa");
outlet(0, "dictionary", myDict.name);
}